Features of Java you must know
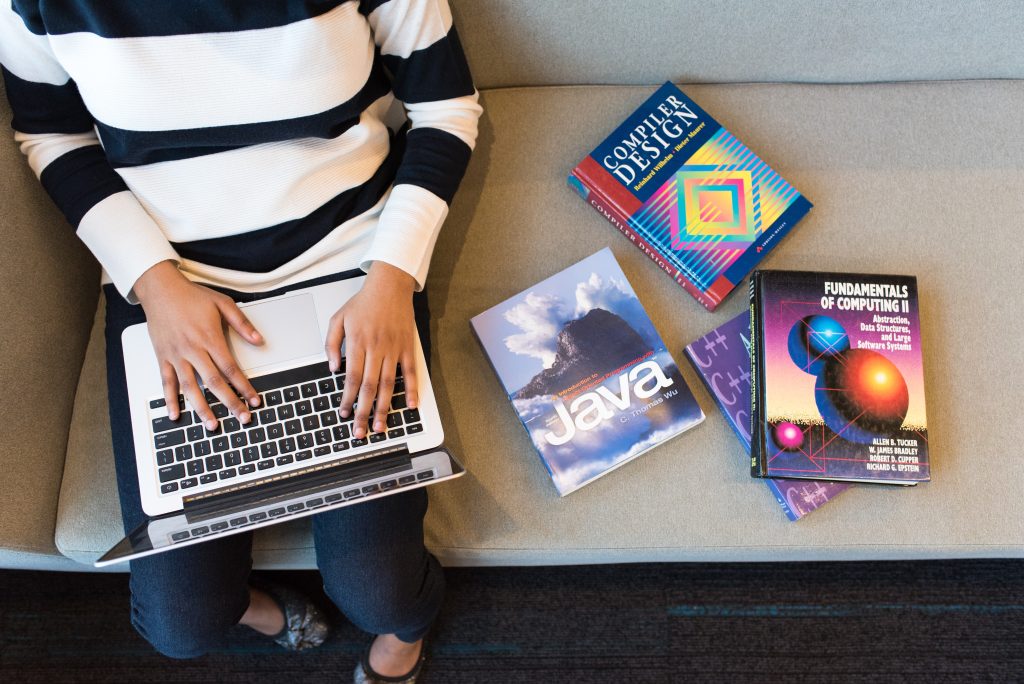
Java is a popular and powerful programming language that has been widely used in software development for over two decades. With its rich set of features, Java enables developers to create robust, scalable, and secure applications. However, to harness the full potential of Java, it is essential to have a good understanding of its key features. In this context, this article will provide an overview of the most important features of java online editor that every programmer should know.
Object-Oriented Programming
Object-Oriented Programming (OOP) is a key feature of Java. Here are some important aspects of OOP in Java:
- Classes and Objects: Java is designed around the concept of classes and objects. A class is a blueprint for an object, and it defines the attributes and behaviors that the object will have. An object is an instance of a class, and it can be created and manipulated at runtime.
- Encapsulation: Encapsulation is the practice of hiding the internal workings of an object from the outside world. In Java, encapsulation is achieved through the use of access modifiers such as private, public, and protected, which control the visibility of a class’s fields and methods.
- Inheritance: Inheritance is the ability of a class to inherit properties and methods from another class. In Java, inheritance is achieved through the use of the extends keyword, which allows a subclass to inherit from a superclass. This feature is useful for creating hierarchies of related classes and for reusing code.
- Polymorphism: Polymorphism is the ability of a single object to take on multiple forms. In Java, polymorphism is achieved through the use of interfaces and abstract classes, which allow different classes to implement the same methods in different ways.
- Abstraction: Abstraction is the practice of simplifying complex systems by breaking them down into smaller, more manageable parts. Inthe java online editor, abstraction is achieved through the use of abstract classes and interfaces, which provide a high-level view of a system without getting into the details of how it works.
OOP in Java is a powerful and flexible feature that allows developers to create complex systems using simple, reusable code. By using classes and objects, encapsulation, inheritance, polymorphism, and abstraction, Java developers can create applications that are easy to understand, maintain, and extend.
Platform independence
One of the key features of Java is its platform independence. This means that Java code can run on any platform that has a Java Virtual Machine (JVM) installed, regardless of the underlying hardware and operating system. Here are some important aspects of the platform-independent feature of Java:
- Bytecode: When you compile a Java program, it is translated into an intermediate language called bytecode. Bytecode is a highly optimized, platform-independent format that can be executed by any JVM. This means that you can write your code once and run it anywhere that has a JVM installed.
- JVM: The JVM is a software program that provides an environment in which Java bytecode can be executed. The JVM is available for a wide range of platforms, including Windows, Linux, macOS, and various mobile operating systems. When you run a Java program, the JVM interprets the bytecode and executes it on the host platform.
- Write Once, Run Anywhere (WORA): The platform-independent feature of Java is often referred to as Write Once, Run Anywhere (WORA). This means that you can write your code on one platform and run it on any other platform that has a JVM installed, without having to modify the code.
- Portability: The platform-independent feature of Java also provides a high degree of portability. This means that you can move your Java code from one platform to another without having to rewrite it. This is particularly useful for large-scale enterprise applications that need to run on a variety of different platforms.
- Security: The platform-independent feature of Java also provides a level of security. Because Java bytecode can be executed on any platform, it is not tied to the underlying hardware or operating system. This makes it more difficult for attackers to exploit vulnerabilities in the system.
In summary, the platform-independent feature of Java is a powerful and important aspect of the language. And it is important for java collections interview questions.
Simple and Familiar
Java has a simple and familiar syntax, which makes it easy for programmers to learn and use. Some of the key features of its syntax are:
- C-like Syntax: Java’s syntax is based on the C programming language, which means that it uses many familiar C-like constructs such as curly braces for blocks of code, semicolons to end statements, and parentheses to define method parameters.
- Object-Oriented Syntax: Java is an object-oriented programming language, so its syntax includes many features that support this paradigm. For example, classes are defined using the “class” keyword, objects are created using the “new” keyword, and methods are called using dot notation.
- Strongly Typed: Java is a strongly typed language, which means that every variable and expression has a specific data type that must be declared before it can be used. This helps prevent errors and ensures that programs are more reliable. It is important for java collections interview questions
Overall, Java’s syntax is designed to be easy to learn and use, while also providing powerful features for building complex applications.
Memory management
Java has automatic memory management, which means that the process of allocating and deallocating memory is managed by the Java Virtual Machine (JVM). This feature makes it easier for programmers to write reliable code without worrying about memory allocation and deallocation.
The memory management features of Java include:
- Heap Memory: In Java, all objects are stored in the heap memory, which is a region of memory allocated to the JVM. The heap memory is managed by the garbage collector, which automatically frees up memory that is no longer in use.
- Garbage Collection: The garbage collector is responsible for freeing up memory that is no longer in use by identifying and removing objects that are no longer referenced by any part of the program. This helps prevent memory leaks and ensures that memory is used efficiently.
- Object Creation: In Java, objects are created using the “new” keyword. When an object is created, the JVM allocates memory for the object on the heap and initializes its state.
Overall, the memory management features of Java help to make programming easier and more reliable by managing memory automatically and preventing common errors.